Realtime Chat app using Kafka, SpringBoot, ReactJS, and WebSockets
In this tutorial, we would be building a simple real-time chat application that demonstrates how to u...
dev.to
0. Kafka, Zookeeper 설치
아래 링크에서 Binary downloads 중 Scala 2.12에 해당하는 압축파일 다운로드
Apache Kafka
Apache Kafka: A Distributed Streaming Platform.
kafka.apache.org
혹은 homebrew를 이용해 설치(Mac OS)
brew install zookeeper
brew install kafka
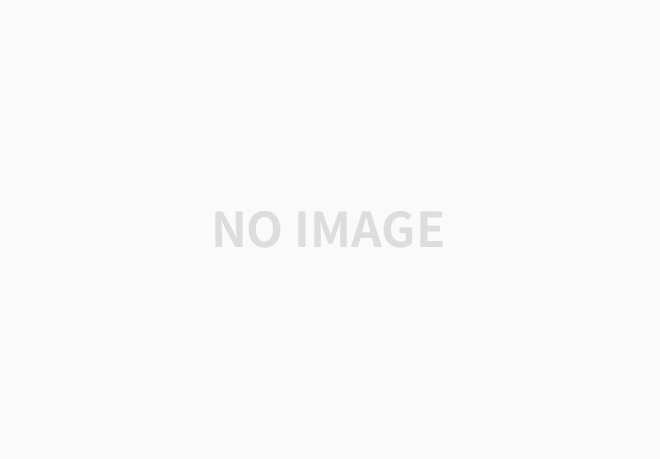
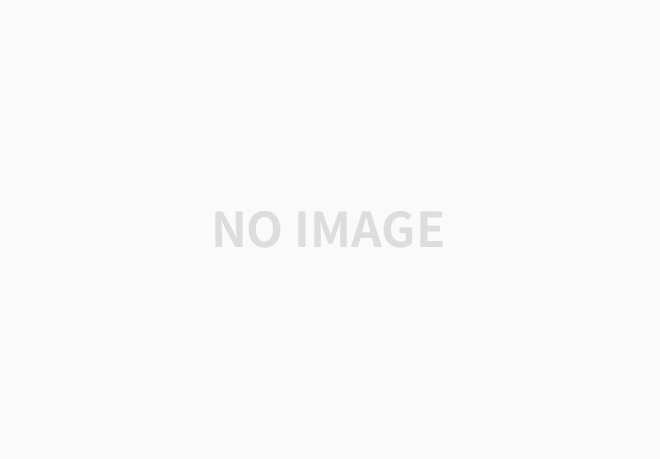
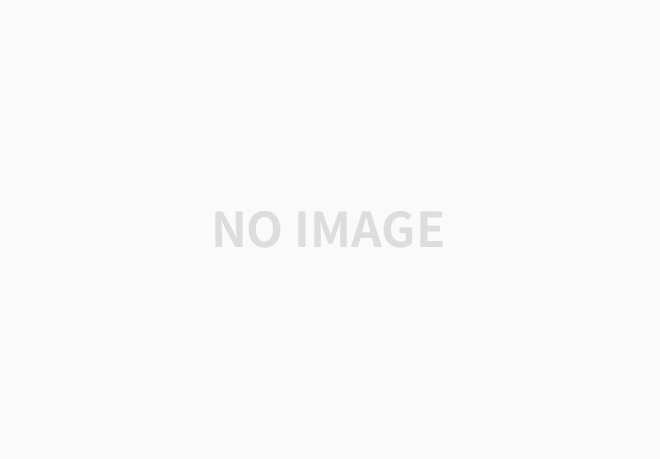
zookeeper서버 시작
zookeeper-server-start /usr/local/etc/kafka/zookeeper.properties
kafka서버 시작
kafka-server-start /usr/local/etc/kafka/server.properties
1. Spring Initializr를 이용해 스프링 부트 프로젝트 생성
원하는대로 선택 및 작성하고, Dependencies에는 Spring for Apache Kafka와 WebSocket을 추가
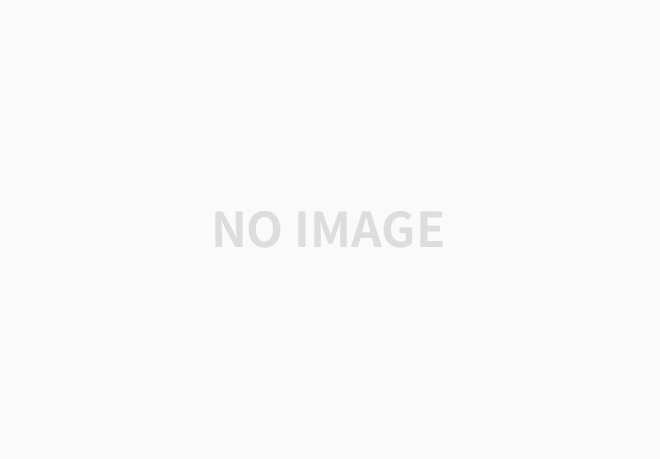
생성 후 압축 해제 > 프로젝트 폴더 경로로 이동 > IDE에서 open
build.gradle
plugins {
id 'org.springframework.boot' version '2.4.1'
id 'io.spring.dependency-management' version '1.0.10.RELEASE'
id 'java'
}
group = 'com.gaemi'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '11'
configurations {
compileOnly {
extendsFrom annotationProcessor
}
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-websocket'
implementation 'org.springframework.kafka:spring-kafka'
compileOnly 'org.projectlombok:lombok'
annotationProcessor 'org.projectlombok:lombok'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
testImplementation 'org.springframework.kafka:spring-kafka-test'
}
test {
useJUnitPlatform()
}
2. Model 작성
메시지를 주고받을 때 사용
기본 생성자 선언 잊지 말기 롬복을 하도 사용했더니 계속 까먹는다..
✔️author - 메시지의 작성자
✔️content - 메시지 내용
✔️timestamp - 메시지가 작성된 시간
public class Message implements Serializable {
private String author;
private String content;
private String timestamp;
public Message() {
}
public Message(String author, String content) {
this.author = author;
this.content = content;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public String getTimestamp() {
return timestamp;
}
public void setTimestamp(String timestamp) {
this.timestamp = timestamp;
}
@Override
public String toString() {
return "Message{" +
"author='" + author + '\'' +
", content='" + content + '\'' +
", timestamp='" + timestamp + '\'' +
'}';
}
}
'STUDY > Spring' 카테고리의 다른 글
Spring Boot | Kafka를 이용한 채팅 (3) 메시지 주고받기 + ReactJS (9) | 2021.01.13 |
---|---|
Spring Boot | Kafka를 이용한 채팅 (2) Kafka 연동 설정 (0) | 2021.01.13 |
Spring Boot | S3파일 업로드 후 CloudFront SignedURL 생성하기 (0) | 2020.12.24 |
Spring Boot | S3 파일 업로드 (0) | 2020.12.22 |
Spring Boot | 스케쥴러(Scheduler) 사용해보기 (0) | 2020.12.21 |